Introduction
Sorting is a crucial concept in data structures. It helps in arranging data in a meaningful order, making searches faster and operations more efficient. One of the simplest sorting algorithms is Bubble Sort. It repeatedly swaps adjacent elements if they are in the wrong order. This process continues until the entire array is sorted.
In this blog post, we will explore the Bubble Sort Algorithm using a function and then implement it manually without a function.
Bubble Sort Using a Function
JavaScript and Python allow functions to simplify tasks. Using a function makes the code reusable and organized. Let’s see how Bubble Sort can be implemented using a function in Python.
def bubble_sort(arr): n = len(arr) for i in range(n): for j in range(0, n - i - 1): if arr[j] > arr[j + 1]: arr[j], arr[j + 1] = arr[j + 1], arr[j] return arr numbers = [64, 34, 25, 12, 22, 11, 90] sorted_numbers = bubble_sort(numbers) print(sorted_numbers)
Explanation
- The function
bubble_sort()
takes an array as input. - It runs nested loops to compare each pair of adjacent elements.
- If the elements are in the wrong order, they are swapped.
- The process continues until no swaps are needed.
- Finally, the sorted array is returned.
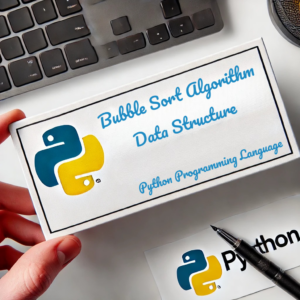
Bubble Sort Without a Function
Now, let’s perform the same logic without using a function. Instead of defining a reusable function, we will write the sorting logic directly in the main program.
numbers = [64, 34, 25, 12, 22, 11, 90] n = len(numbers) for i in range(n): for j in range(0, n - i - 1): if numbers[j] > numbers[j + 1]: numbers[j], numbers[j + 1] = numbers[j + 1], numbers[j] print(numbers)
Explanation
- The logic remains the same.
- Instead of calling a function, we write the sorting steps directly inside the program.
- The numbers are compared, swapped, and finally printed in ascending order.
Comparison Between Both Approaches
Feature | Using Function | Without Function |
---|---|---|
Readability | High | Moderate |
Reusability | Yes | No |
Maintainability | Easy | Difficult |
Flexibility | More | Less |
Using a function improves reusability. Without a function, the logic is straightforward but less maintainable.
Conclusion
Bubble Sort is a simple sorting algorithm that works by repeatedly swapping elements. When implemented using a function, it becomes more structured. When written without a function, it is direct but harder to maintain. Regardless of the approach, mastering sorting techniques is essential for data structure learning.