Unique Python Logic – Finding the Largest Word in a Sentence (With and Without Functions)
Python provides a variety of built-in functions that make coding easier. However, sometimes solving a problem without built-in functions helps improve logical thinking. In this blog, we will explore how to find the largest word in a sentence, both using a built-in function and without any functions.
Finding the Largest Word in a Sentence Using Python’s Built-in Functions
Python’s built-in functions like max()
make it easy to find the longest word in a sentence.
Code Example (Using Built-in Functions)
def find_largest_word(sentence): words = sentence.split() # Splitting sentence into words largest_word = max(words, key=len) # Finding the longest word return largest_word # Example Usage sentence = "Python programming is both fun and powerful" print("Largest Word:", find_largest_word(sentence))
Explanation:
- The sentence is split into individual words using
split()
. - The
max()
function is used withkey=len
to find the word with the maximum length. - The function returns the longest word.
Output:
Largest Word: programming
This method is efficient, readable, and Pythonic. But what if we solve this without using built-in functions like max()
? Let’s explore that next.
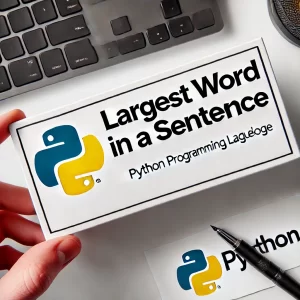
Finding the Largest Word Without Any Built-in Functions
Here, we implement the logic manually, without relying on functions like max()
or split()
.
Code Example (Without Built-in Functions)
sentence = "Python programming is both fun and powerful" # Manual logic without max() function words = [] word = "" for char in sentence: if char != " ": word += char # Building words character by character else: words.append(word) # Adding words to the list word = "" words.append(word) # Adding the last word # Finding the longest word manually largest_word = "" for w in words: if len(w) > len(largest_word): largest_word = w print("Largest Word:", largest_word)
Explanation:
- Instead of using
split()
, we manually separate words by checking spaces. - Each word is stored in a list.
- A loop checks for the longest word manually.
Output:
Largest Word: programming
Even without built-in functions, we can achieve the same result using logical steps. This approach is useful when working in environments with restricted libraries.
Why Learn Both Methods?
- Efficiency – Built-in functions are optimized and faster.
- Problem-Solving Skills – Writing logic manually improves understanding.
- Flexibility – Knowing both methods allows coding in different scenarios.
For beginners, learning built-in functions saves time, while manual logic improves problem-solving skills. By practicing both, you become a better Python programmer.